SpringBoot整合JSP和Shiro避坑!
SpringBoot整合JSP Shiro示例
今天在使用SpringBoot项目中整合Shiro框架时,使用JSP页面作为前端页面,不料在SpringBoot整合JSP时,遇到一些问题,在排除问题时,针对于Shiro框架的使用有了一些新的了解,在这里就简单记录下。
1.整合JSP
1.1 新建SpringBoot项目
我这里使用的是目前最新的版本SpringBoot2.6.7
。
1.2 添加依赖坐标
<dependencies>
<!--引入jsp解析依赖-->
<!-- 以下两个依赖非必选 -->
<!--<dependency>-->
<!-- <groupId>javax.servlet</groupId>-->
<!-- <artifactId>jstl</artifactId>-->
<!--</dependency>-->
<!-- Provided 编译和测试的时候使用-->
<!--<dependency>-->
<!-- <groupId>org.springframework.boot</groupId>-->
<!-- <artifactId>spring-boot-starter-tomcat</artifactId>-->
<!-- <scope>provided</scope>-->
<!--</dependency>-->
<!-- 对jsp的支持的依赖 必须添加-->
<dependency>
<groupId>org.apache.tomcat.embed</groupId>
<artifactId>tomcat-embed-jasper</artifactId>
<scope>provided</scope>
</dependency>
<!--web环境-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!--测试环境-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
注意:在这里关于引入JSP相关的依赖坐标,网络上有许多各种各样的答案,但是在我当前的项目测试下,有的是不能正常运行的,在这里只给出了以上可以正常运行应用的,至少在我这里可以跑起来。经过我的尝试发现,tomcat-embed-jasper
是必须要添加的坐标,但是jstl
、spring-boot-starter-tomcat
不添加时,项目也可以正常运行。还有注意这里jstl
的groupId
是javax.servlet
。如果需要详细学习可以去网上查查相关资料,我这里不怎么用JSP,也就不太了解。
1.3 项目配置
spring:
mvc:
view:
prefix: /WEB-INF/jsp/
suffix: .jsp
很显然,这是Spring MVC的视图相关的配置,通过配置发现,我们需要去项目添加webapp
目录。
1.4 添加webapp目录
我这里就简单将全部操作放到了一张图,根据标号进行操作即可!
注意:\webapp\WEB-INF\web.xml
在项目中的路径!
以我这里为例:springbootShiro
为项目名。
C:\Users\Smile\Desktop\shrio\springbootShiro\src\main\webapp\WEB-INF\web.xml
即在项目的src\main目录下!
完成后项目结构如下:
1.5 添加index.jsp
<%--
Created by IntelliJ IDEA.
User: Smile
Date: 2022/5/6
Time: 21:07
To change this template use File | Settings | File Templates.
--%>
<%@ page isELIgnored="false" %>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>Title</title>
</head>
<body>
<h1>我是JSP页面</h1>
</body>
</html>
注意:是在WEB-INF目录下新建jsp文件夹,在jsp内添加jsp文件,与上面的项目配置相关的路径保持一致。
1.6 编写Controller
/**
* @Classname HelloController
* @Description TODO
* @Date 2022/4/7 10:28
* @Created by YJS
* @WebSite www.imyjs.cn
*/
@Controller
public class HelloController {
@RequestMapping("/")
public String hello(){
return "index";
}
}
1.7 测试
在浏览器中访问路径:http://localhost:8080/
2.整合Shiro
下面接着上边的项目进行整合。
2.1 添加依赖坐标
在上边的基础之上,继续添加如下依赖坐标:
<dependencies>
<!--Mysql-->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
</dependency>
<!--数据库连接池-->
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>druid-spring-boot-starter</artifactId>
<version>1.2.6</version>
</dependency>
<!--Mybatis-Plus-->
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.5.1</version>
</dependency>
<!--Shrio安全框架-->
<!--报错:No bean of type 'org.apache.shiro.realm.Realm' found.-->
<!--Please create bean of type 'Realm' or add a shiro.ini in the root classpath
(src/main/resources/shiro.ini) or in the META-INF folder
(src/main/resources/META-INF/shiro.ini).
-->
<dependency>
<groupId>org.apache.shiro</groupId>
<artifactId>shiro-spring-boot-starter</artifactId>
<version>1.8.0</version>
</dependency>
</dependencies>
注意:在添加数据库连接池坐标后需要进行数据源相关的配置才能正常运行应用,Shrio安全框架在这里也有类似的坑!
2.2 修改项目配置
server:
port: 80
spring:
mvc:
view:
prefix: /WEB-INF/jsp/
suffix: .jsp
datasource:
druid:
driver-class-name: com.mysql.cj.jdbc.Driver
url: jdbc:mysql://localhost:3306/shrio?characterEncoding=utf-8&useSSL=false&serverTimezone=GMT
username: root
password: 12313
mybatis-plus:
global-config:
db-config:
table-prefix: tb_
添加数据源相关配置等。
2.3 Shiro框架避坑
经过以上操作后启动项目,会抛出如下异常:
No bean of type 'org.apache.shiro.realm.Realm' found.
Please create bean of type 'Realm' or add a shiro.ini in the root classpath
(src/main/resources/shiro.ini) or in the META-INF folder(src/main/resources/META-INF/shiro.ini).
好像是需要Realm,于是就在项目的classpath类路径下添加一个shiro.ini
文件,注意空文件无效,需要添加内容而且按照他的格式,以下示例:
[users]
admin=123456
2.4 测试
此时访问路径,如果页面打不开,并且地址栏内路径自动跳转到项目index.jsp时,就表示Shrio已经成功配置。
3.总结
一阵操作下来,回头一看其实并不难,但是在整个寻找问题解决问题的过程中确是各种焦虑,遇到问题一定要冷静,切记不要一闷头的去search、不管不顾的各种Copy,结果问题没解决,反而搞丢了心态。在解决问题时,可以从头开始保持一个比较干净的环境,避免问题的源头过多,这样不容易排查问题,并且每走一步就进行及时的测试,多注意阅读异常信息,大多时候可以直接找到问题的根源。
走的慢才能进步的快!共勉!
微信关注
编程那点事儿
本站所发布的一切软件资源、文章内容、页面内容可能整理来自于互联网,在此郑重声明本站仅限用于学习和研究目的;并告知用户不得将上述内容用于商业或者非法用途,否则一切后果请用户自负。
如果本站相关内容有侵犯到您的合法权益,请仔细阅读本站公布的投诉指引页相关内容联系我,依法依规进行处理!
作者:理想
链接:https://www.imyjs.cn/archives/773

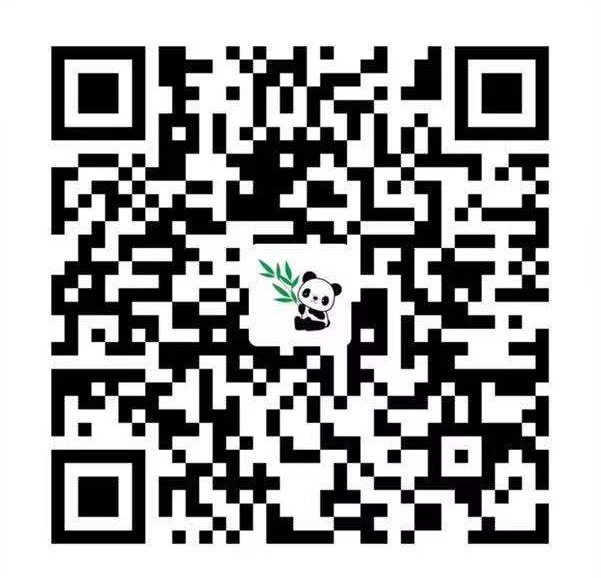
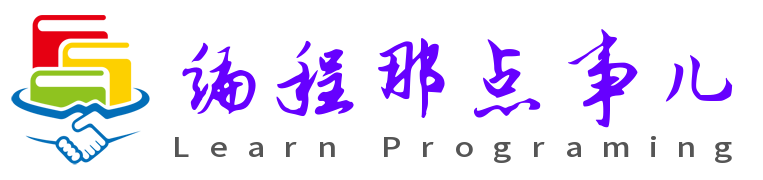

共有 0 条评论