Java 操作PDF图片合并、PDF图片互转
导入所需依赖jar坐标
<dependencies>
<!-- https://mvnrepository.com/artifact/com.itextpdf/itextpdf -->
<dependency>
<groupId>com.itextpdf</groupId>
<artifactId>itextpdf</artifactId>
<version>5.5.13</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.apache.pdfbox/pdfbox -->
<dependency>
<groupId>org.apache.pdfbox</groupId>
<artifactId>pdfbox</artifactId>
<version>2.0.24</version>
</dependency>
</dependencies>
PDF与图片合并
public class Main {
public static void main(String[] args) throws IOException, DocumentException {
// 读入PDF文档
File file = new File("a.pdf");
// 合成后的PDF文档
FileOutputStream outputStream = new FileOutputStream("c.pdf");
PdfReader reader = new PdfReader(new FileInputStream(file));
PdfStamper stamper = new PdfStamper(reader, outputStream);
// 将签名图片放在pdf文件的第2页
PdfContentByte over = stamper.getOverContent(2);
// 签名图片
Image contractSealImg = Image.getInstance("c.png");
over.saveState();
PdfGState pdfGState = new PdfGState();
// 设置图片的透明度
// pdfGState.setFillOpacity(0.8F);
over.setGState(pdfGState);
// 设置图片位置
contractSealImg.setAbsolutePosition(480,650);
// 设置图片大小
contractSealImg.scaleAbsolute(80, 60);
// 将图片添加到PDF文档
over.addImage(contractSealImg);
over.restoreState();
stamper.setFormFlattening(true);
stamper.close();
reader.close();
outputStream.close();
}
}
PDF转换成图片
public class PDF2PNG {
public static void main(String[] args) {
try {
// 加载PDF文件
PDDocument document = PDDocument.load(new File("c.pdf"));
// 创建PDFRenderer对象
PDFRenderer renderer = new PDFRenderer(document);
// 获取PDF文件的页数
int pageCount = document.getNumberOfPages();
// 循环处理每一页
for (int i = 0; i < pageCount; i++) {
// 渲染当前页为图像
BufferedImage image = renderer.renderImage(i);
// 将图像保存为PNG文件
ImageIO.write(image, "PNG", new File("output" + i + ".png"));
}
// 关闭PDF文件
document.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
图片转换PDF
public class ImgToPdfUtil {
public static File Pdf(ArrayList<String> imageUrllist, String mOutputPdfFileName) {
// new一个新的PDF文档
Document doc = new Document(PageSize.A4, 0, 0, 0, 0);
try {
// pdf写入
PdfWriter.getInstance(doc, new FileOutputStream(mOutputPdfFileName));
// 打开文档
doc.open();
// 循环图片List,将图片加入到pdf中
for (int i = 0; i < imageUrllist.size(); i++) {
// 在pdf创建一页
doc.newPage();
// 通过文件路径获取image
Image png1 = Image.getInstance(imageUrllist.get(i));
float heigth = png1.getHeight();
float width = png1.getWidth();
int percent = getPercent2(heigth, width);
png1.setAlignment(Image.MIDDLE);
// 表示是原来图像的比例;
png1.scalePercent(percent + 3);
doc.add(png1);
}
doc.close();
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (DocumentException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
// 输出流
File mOutputPdfFile = new File(mOutputPdfFileName);
if (!mOutputPdfFile.exists()) {
mOutputPdfFile.deleteOnExit();
return null;
}
// 反回文件输出流
return mOutputPdfFile;
}
public static int getPercent(float h, float w) {
int p = 0;
float p2 = 0.0f;
if (h > w) {
p2 = 297 / h * 100;
} else {
p2 = 210 / w * 100;
}
p = Math.round(p2);
return p;
}
public static int getPercent2(float h, float w) {
int p = 0;
float p2 = 0.0f;
p2 = 530 / w * 100;
p = Math.round(p2);
return p;
}
public void imgOfPdf(String filepath, String imgUrl) {
try {
// 图片list集合
ArrayList<String> imageUrllist = new ArrayList<String>();
String[] imgUrls = imgUrl.split(",");
for (int i=0; i<imgUrls.length; i++) {
imageUrllist.add(imgUrls[i]);
}
// 输出pdf文件路径
String pdfUrl = filepath;
// 生成pdf
File file = Pdf(imageUrllist, pdfUrl);
file.createNewFile();
} catch (IOException e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
ImgToPdfUtil img = new ImgToPdfUtil();
img.imgOfPdf("hkxx.pdf","hkxx.png");
}
}
微信关注
编程那点事儿
本站为非盈利性站点,所有资源、文章等仅供学习参考,并不贩卖软件且不存在任何商业目的及用途,如果您访问和下载某文件,表示您同意只将此文件用于参考、学习而非其他用途。
本站所发布的一切软件资源、文章内容、页面内容可能整理来自于互联网,在此郑重声明本站仅限用于学习和研究目的;并告知用户不得将上述内容用于商业或者非法用途,否则一切后果请用户自负。
如果本站相关内容有侵犯到您的合法权益,请仔细阅读本站公布的投诉指引页相关内容联系我,依法依规进行处理!
作者:理想
链接:https://www.imyjs.cn/archives/1321
本站所发布的一切软件资源、文章内容、页面内容可能整理来自于互联网,在此郑重声明本站仅限用于学习和研究目的;并告知用户不得将上述内容用于商业或者非法用途,否则一切后果请用户自负。
如果本站相关内容有侵犯到您的合法权益,请仔细阅读本站公布的投诉指引页相关内容联系我,依法依规进行处理!
作者:理想
链接:https://www.imyjs.cn/archives/1321
THE END
0
二维码

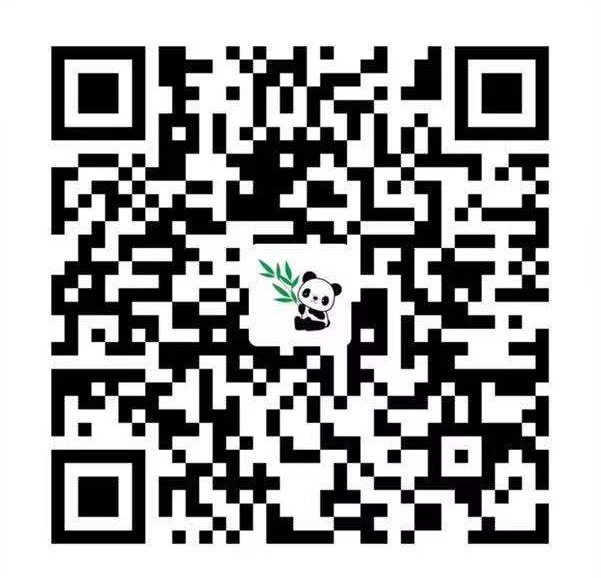
Java 操作PDF图片合并、PDF图片互转
导入所需依赖jar坐标
<dependencies>
……
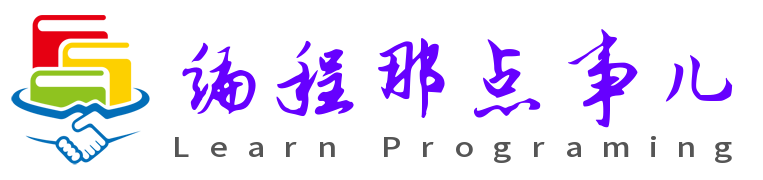
文章目录
关闭

共有 0 条评论