一篇文章学会快速使用Mybatis!
一、Mybatis简介
MyBatis 是一款优秀的持久层框架
,用于简化 JDBC 开发
官网:https://mybatis.org/mybatis-3/zh/index.html
持久层:
- 负责将数据到保存到数据库的那一层代码。
以后开发我们会将操作数据库的Java代码作为持久层。而Mybatis就是对jdbc代码进行了封装。
- JavaEE三层架构:表现层、业务层、持久层
二、Mybatis快速入门
1.创建模块导入坐标
在创建好的模块中的 pom.xml 配置文件中添加依赖的坐标
<dependencies>
<!--mybatis 依赖-->
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis</artifactId>
<version>3.5.5</version>
</dependency>
<!--mysql 驱动-->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.46</version>
</dependency>
<!--junit 单元测试-->
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.13</version>
<scope>test</scope>
</dependency>
<!-- 添加slf4j日志api -->
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-api</artifactId>
<version>1.7.20</version>
</dependency>
<!-- 添加logback-classic依赖 -->
<dependency>
<groupId>ch.qos.logback</groupId>
<artifactId>logback-classic</artifactId>
<version>1.2.3</version>
</dependency>
<!-- 添加logback-core依赖 -->
<dependency>
<groupId>ch.qos.logback</groupId>
<artifactId>logback-core</artifactId>
<version>1.2.3</version>
</dependency>
</dependencies>
注意:需要在项目的 resources 目录下创建logback的配置文件
<?xml version="1.0" encoding="UTF-8"?>
<configuration>
<!--
CONSOLE :表示当前的日志信息是可以输出到控制台的。
-->
<appender name="Console" class="ch.qos.logback.core.ConsoleAppender">
<encoder>
<pattern>[%level] %cyan([%thread]) %boldGreen(%logger{15}) - %msg %n</pattern>
</encoder>
</appender>
<logger name="cn.imyjs" level="DEBUG" additivity="false">
<appender-ref ref="Console"/>
</logger>
<!--
level:用来设置打印级别,大小写无关:TRACE, DEBUG, INFO, WARN, ERROR, ALL 和 OFF
, 默认debug
<root>可以包含零个或多个<appender-ref>元素,标识这个输出位置将会被本日志级别控制。
-->
<root level="DEBUG">
<appender-ref ref="Console"/>
</root>
</configuration>
2.编写核心配置文件
在模块下的 resources 目录下创建mybatis的配置文件 mybatis-config.xml
,内容如下:
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE configuration
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<typeAliases>
<package name="cn.imyjs.pojo"/>
</typeAliases>
<!--
environments:配置数据库连接环境信息。可以配置多个environment,通过default属性切换不同的environment
-->
<environments default="development">
<environment id="development">
<transactionManager type="JDBC"/>
<dataSource type="POOLED">
<!--数据库连接信息-->
<property name="driver" value="com.mysql.jdbc.Driver"/>
<property name="url" value="jdbc:mysql://127.0.0.1:3306/db1?rewriteBatchedStatements=true&characterEncoding=UTF-8&useSSL=false"/>
<property name="username" value="root"/>
<property name="password" value="0000000"/>
</dataSource>
</environment>
</environments>
<mappers>
<!--加载sql映射文件-->
<!-- <mapper resource="cn/imyjs/mapper/UserMapper.xml"/>-->
<!--Mapper代理方式-->
<package name="cn.imyjs.mapper"/>
</mappers>
</configuration>
3.编写 SQL 映射文件
在模块的 resources
目录下创建映射配置文件 UserMapper.xml
,内容如下:
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="test">
<select id="selectAll" resultType="cn.imyjs.pojo.User">
select * from tb_user;
</select>
</mapper>
4.编码
4.1编写pojo类
在 cn.imyjs.pojo
包下创建 User类
public class User {
private int id;
private String username;
private String password;
private String gender;
private String addr;
//省略了 setter 和 getter
}
4.2编写MybatisDemo类
public class MyBatisDemo {
public static void main(String[] args) throws IOException {
//1. 加载mybatis的核心配置文件,获取 SqlSessionFactory
String resource = "mybatis-config.xml";
InputStream inputStream = Resources.getResourceAsStream(resource);
SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(inputStream);
//2. 获取SqlSession对象,用它来执行sql
SqlSession sqlSession = sqlSessionFactory.openSession();
//3. 执行sql
List<User> users = sqlSession.selectList("test.selectAll"); //参数是一个字符串,该字符串必须是映射配置文件的namespace.id
System.out.println(users);
//4. 释放资源
sqlSession.close();
}
}
三、Mapper代理开发
1.使用Mapper代理要求
使用Mapper代理方式,必须满足以下要求:
- 定义与SQL映射文件
同名
的Mapper接口,并且将Mapper接口和SQL映射文件放置在同一目录
下。 - 设置SQL映射文件的namespace属性为Mapper接口
全限定名
<mapper namespace="cn.imyjs.mapper.BrandMapper"> </mapper>
- 在 Mapper 接口中定义方法,方法名就是SQL映射文件中sql语句的id,并保持参数类型和返回值类型一致
public interface BrandMapper { List<Brand> selectAll(); Brand selectById(int id); }
<select id="selectAll" resultType="cn.imyjs.pojo.Brand"> select * from tb_brand; </select> <select id="selectById" parameterType="int" resultType="cn.imyjs.pojo.Brand"> select * from tb_brand where id = #{id}; </select>
2.注意
如果Mapper接口名称和SQL映射文件名称相同,并在同一目录下,则可以使用包扫描的方式简化SQL映射文件的加载。也就是将核心配置文件的加载映射配置文件
的配置修改为
<mappers>
<!--加载sql映射文件-->
<!-- <mapper resource="cn/imyjs/mapper/UserMapper.xml"/>-->
<!--Mapper代理方式-->
<package name="cn.imyjs.mapper"/>
</mappers>
3.类型别名
在映射配置文件中的 resultType
属性需要配置数据封装的类型(类的全限定名)。而每次这样写是特别麻烦的,Mybatis 提供了 类型别名
(typeAliases) 可以简化这部分的书写。
首先需要现在核心配置文件
中配置类型别名,也就意味着给pojo包下所有的类起了别名(别名就是类名),不区分大小写
。内容如下:
<typeAliases>
<!--name属性的值是实体类所在包-->
<package name="cn.imyjs.pojo"/>
</typeAliases>
通过上述的配置,我们就可以简化映射配置文件中 resultType
属性值的编写
<--配置别名前:-->
<select id="selectAll" resultType="cn.imyjs.pojo.Brand">
select *
from tb_brand;
</select>
<--配置别名后:-->
<select id="selectAll" resultType="brand">
select *
from tb_brand;
</select>
四、动态SQL
Mybatis对动态SQL有很强大的支撑:
- if
- choose (when, otherwise)
- trim (where, set)
- foreach
if 标签
if 标签用于条件判断
,test 属性
:逻辑表达式
<select id="selectByCondition" resultMap="brandResultMap">
select *
from tb_brand
where
<if test="status != null">
and status = #{status}
</if>
<if test="companyName != null and companyName != '' ">
and company_name like #{companyName}
</if>
<if test="brandName != null and brandName != '' ">
and brand_name like #{brandName}
</if>
</select>
如上的这种SQL语句就会根据传递的参数值进行动态的拼接。如果此时status和companyName有值那么就会值拼接这两个条件。
但是它也存在问题,如果此时给的参数值是
Map map = new HashMap();
// map.put("status" , status);
map.put("companyName", companyName);
map.put("brandName" , brandName);
拼接的SQL语句就变成了
select * from tb_brand where and company_name like ? and brand_name like ?
而上面的语句中where 关键后直接跟 and 关键字
,这就是一条错误的SQL语句。这个就可以使用 where 标签解决
where 标签
作用:
- 替换where关键字
- 会动态的去掉第一个条件前的 and
- 如果所有的参数没有值则不加where关键字
<select id="selectByCondition" resultMap="brandResultMap">
select *
from tb_brand
<where>
<if test="status != null">
and status = #{status}
</if>
<if test="companyName != null and companyName != '' ">
and company_name like #{companyName}
</if>
<if test="brandName != null and brandName != '' ">
and brand_name like #{brandName}
</if>
</where>
</select>
注意:需要给每个条件前都加上 and 关键字。
choose标签
在查询时只能选择 品牌名称
、当前状态
、企业名称
这三个条件中的一个,但是用户到底选择哪儿一个,我们并不能确定。这种就属于单个条件的动态SQL语句。
这种需求需要使用到 choose(when,otherwise)标签
实现, 而 choose
标签类似于Java 中的switch语句。
在 BrandMapper.xml
映射配置文件中编写 statement
,使用 resultMap
而不是使用 resultType
<select id="selectByConditionSingle" resultMap="brandResultMap">
select *
from tb_brand
<where>
<choose><!--相当于switch-->
<when test="status != null"><!--相当于case-->
status = #{status}
</when>
<when test="companyName != null and companyName != '' "><!--相当于case-->
company_name like #{companyName}
</when>
<when test="brandName != null and brandName != ''"><!--相当于case-->
brand_name like #{brandName}
</when>
</choose>
</where>
</select>
set 标签
set 标签可以用于动态包含需要更新的列,忽略其它不更新的列。
<update id="update">
update tb_brand
<set>
<if test="brandName != null and brandName != ''">
brand_name = #{brandName},
</if>
<if test="companyName != null and companyName != ''">
company_name = #{companyName},
</if>
<if test="ordered != null">
ordered = #{ordered},
</if>
<if test="description != null and description != ''">
description = #{description},
</if>
<if test="status != null">
status = #{status}
</if>
</set>
where id = #{id};
</update>
foreach 标签
用来迭代任何可迭代的对象(如数组,集合)。
- collection 属性:
- mybatis会将数组参数,封装为一个Map集合。
- 默认:array = 数组
- 使用@Param注解改变map集合的默认key的名称
- mybatis会将数组参数,封装为一个Map集合。
- item 属性:本次迭代获取到的元素。
- separator 属性:集合项迭代之间的分隔符。
foreach
标签不会错误地添加多余的分隔符。也就是最后一次迭代不会加分隔符。 - open 属性:该属性值是在拼接SQL语句之前拼接的语句,只会拼接一次
- close 属性:该属性值是在拼接SQL语句拼接后拼接的语句,只会拼接一次
<delete id="deleteByIds">
delete from tb_brand where id
in
<foreach collection="array" item="id" separator="," open="(" close=")">
#{id}
</foreach>
;
</delete>
假如数组中的id数据是{1,2,3},那么拼接后的sql语句就是:
delete from tb_brand where id in (1,2,3);
五、Mybatis SQL语句常见问题
1.对象属性与表属性不一致
这个问题可以通过下面方式进行解决:
1.1给字段起别名
我们可以在写sql语句时给这两个字段起别名,将别名定义成和属性名一致即可。
<mapper namespace="cn.imyjs.mapper.BrandMapper">
<select id="selectAll" resultType="brand">
select id, brand_name as brandName, company_name as companyName, ordered, description, status
from tb_brand;
</select>
</mapper>
缺点:如果表中还有更多的字段将导致书写麻烦,同时其他的功能也需要查询这些字段时就显得我们的代码不够精炼。
解决办法:Mybatis提供了sql
片段可以提高sql的复用性。
1.2SQL片段
- 将需要复用的SQL片段抽取到
sql
标签中<sql id="brand_column"> id, brand_name as brandName, company_name as companyName, ordered, description, status </sql>
id属性值是唯一标识,引用时也是通过该值进行引用
。 - 在sql语句中进行引用
使用
include
标签引用上述的 SQL 片段,而refid
指定上述 SQL 片段的id值。<select id="selectAll" resultType="brand"> select <include refid="brand_column" /> from tb_brand; </select>
缺点:如果还有功能只需要查询部分字段,而不是查询所有字段,那么我们就需要再定义一个 SQL 片段,这就显得不是那么灵活。
解决办法:使用resultMap来定义字段和属性的映射关系的方式解决上述问题。
1.3使用resultMap定义映射关系
- 在映射配置文件中使用resultMap定义
字段
和属性
的映射关系<resultMap id="brandResultMap" type="brand"> <!-- id:完成主键字段的映射 column:表的列名 property:实体类的属性名 result:完成一般字段的映射 column:表的列名 property:实体类的属性名 --> <result column="brand_name" property="brandName"/> <result column="company_name" property="companyName"/> </resultMap>
注意:在上面只需要定义 字段名 和 属性名 不一样的映射,而一样的则不需要专门定义出来。
- SQL语句resultType 修改为 resultMap
<select id="selectAll" resultMap="brandResultMap"> select * from tb_brand; </select>
2.参数占位符
Mybatis提供了两种参数占位符:
#{}
:执行SQL时,会将 #{} 占位符替换为?,将来自动设置参数值。从上述例子可以看出使用#{} 底层使用的是PreparedStatement
${}
:拼接SQL。底层使用的是Statement
,会存在SQL注入问题。
以后开发我们使用 #{} 参数占位符。
模糊查询
MyBatis:模糊查询的4种实现方式:MyBatis:模糊查询的4种实现方式 - 学编程那点事儿 (imyjs.cn)
3.SQL语句中特殊字段处理
转义字符
在xml中有5个预定义的实体引用(entity reference):
特殊符号 | 实体引用 | XML中的含义 | |
---|---|---|---|
< | < | 新元素的开始 | 小于号 |
> | > | 大于号 | |
& | & | 字符实体的开始 | 逻辑与 |
" | " | 双引号 | |
' | &apos | 单引号 |
注意:只有"<"和"&",对于xml来说是严格禁止使用的。引号和大于号是合法的,但是把它们替换为实体引用是个好的习惯。
使用<![CDATA[内容]]>
select *
from tb_brand
where id <![CDATA[
<
]]> #{id};
4.Mybatis多参数实现
4.1 @Param("参数名称")
使用 @Param("参数名称")
标记每一个参数,在映射配置文件中就需要使用 #{参数名称}
进行占位
List<Brand> selectByCondition(@Param("status") int status, @Param("companyName") String companyName,@Param("brandName") String brandName);
4.2封装成一个实体对象
将多个参数封装成一个 实体对象 ,将该实体对象作为接口的方法参数。该方式要求在映射配置文件的SQL中使用 #{内容}
时,里面的内容必须和实体类属性名保持一致
。
List<Brand> selectByCondition(Brand brand);
4.3封装到map集合
将多个参数封装到map集合中,将map集合作为接口的方法参数。该方式要求在映射配置文件的SQL中使用 #{内容}
时,里面的内容必须和map集合中键的名称一致
。
List<Brand> selectByCondition(Map map);
六、注解实现CRUD
Mybatis 针对 CURD 操作都提供了对应的注解,已经做到见名知意。如下:
- 查询 :@Select
- 添加 :@Insert
- 修改 :@Update
- 删除 :@Delete
注解是用来替换映射配置文件方式配置的,所以使用了注解,就不需要再映射配置文件中书写对应的
注解完成简单功能,配置文件完成复杂功能。
@Select(value = "select * from tb_user where id = #{id}")
public User select(int id);
微信关注
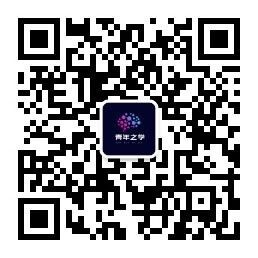